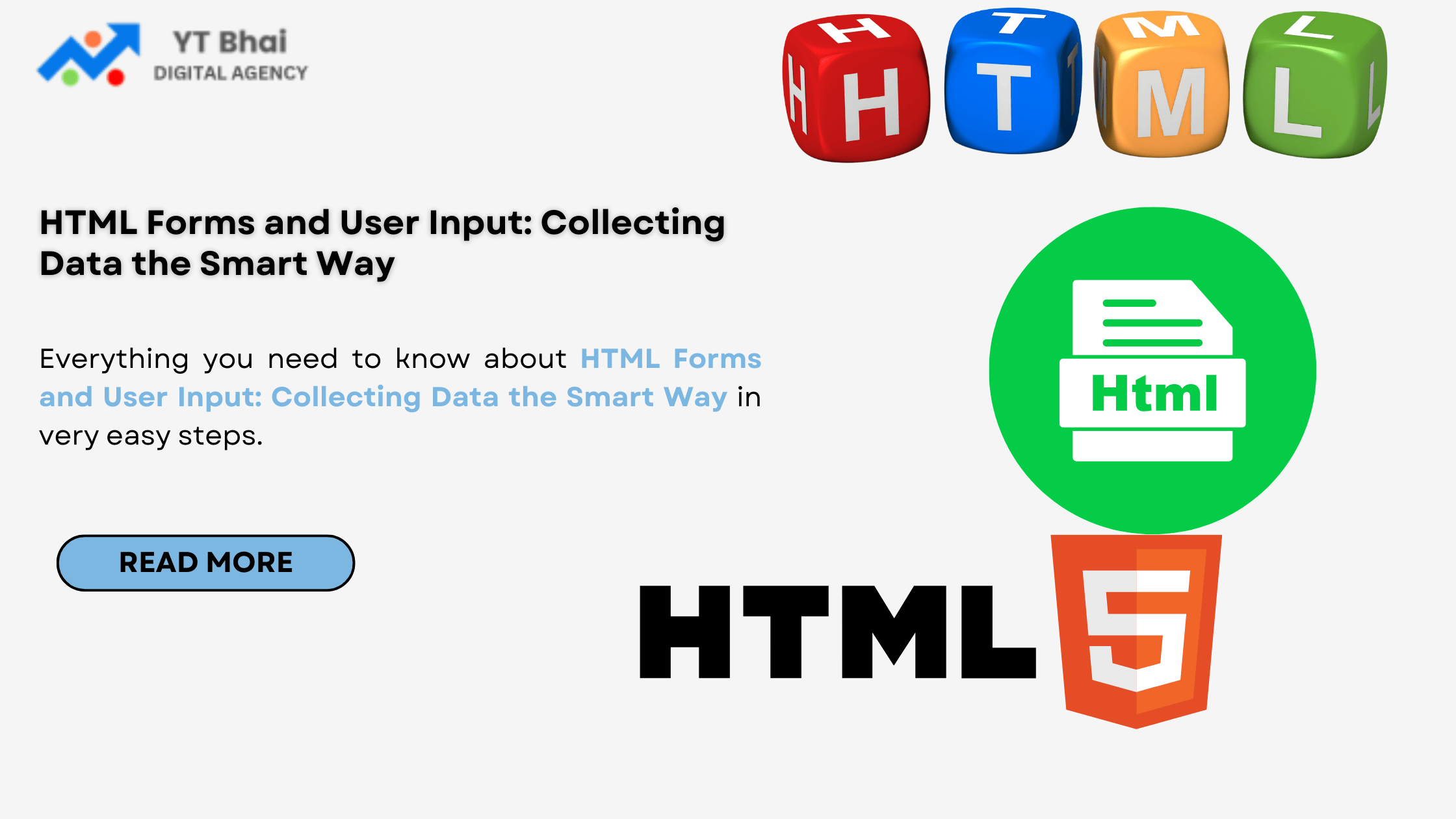
Forms are a crucial part of web development, enabling users to interact with websites by providing input or submitting data. Whether it’s signing up for a newsletter, placing an order, or leaving feedback, HTML forms serve as the bridge between users and web servers. In this blog, we’ll delve into the basics of HTML forms, exploring their structure, essential elements, and how they work.
What Are HTML Forms?
An HTML form is a section of a web page that collects user input. Forms use various input fields, buttons, and other elements to gather data and submit it to a server for processing.
Key Features:
- Data Collection: Forms collect user data such as text, passwords, and file uploads.
- Server Communication: Data is sent to a server for processing using methods like GET or POST.
- Customizable Input: Developers can use various input types and validation techniques.
Here’s an example of a simple HTML form:
<form action="submit.php" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
Basic Structure of an HTML Form
Every form has the following core components:
1. The <form>
Tag
Defines the start and end of a form.
action
: Specifies where the form data should be sent (e.g., a server-side script).method
: Specifies the HTTP method (GET or POST) to use when sending data.
2. Input Elements
These fields allow users to enter data. Common input types include:
Text Input:
<input type="text" name="username" placeholder="Enter your name">
Password Input:
<input type="password" name="password" placeholder="Enter your password">
Email Input:
<input type="email" name="email" placeholder="Enter your email">
File Upload:
<input type="file" name="file">
3. Labels
Labels improve form accessibility by associating text with input fields:
<label for="email">Email Address:</label>
<input type="email" id="email" name="email">
4. Buttons
Used to submit or reset a form:
- Submit Button:
<input type="submit" value="Submit">
- Reset Button:
<input type="reset" value="Clear">
Common Form Elements and Attributes
1. Dropdown Menus (<select>
):
Allow users to choose from predefined options:
<label for="country">Choose your country:</label>
<select id="country" name="country">
<option value="us">United States</option>
<option value="uk">United Kingdom</option>
<option value="in">India</option>
</select>
2. Radio Buttons:
Enable users to select one option from a group:
<p>Gender:</p>
<input type="radio" id="male" name="gender" value="male">
<label for="male">Male</label>
<input type="radio" id="female" name="gender" value="female">
<label for="female">Female</label>
3. Checkboxes:
Allow multiple selections:
<p>Select your hobbies:</p>
<input type="checkbox" id="hobby1" name="hobby" value="reading">
<label for="hobby1">Reading</label>
<input type="checkbox" id="hobby2" name="hobby" value="traveling">
<label for="hobby2">Traveling</label>
4. Textarea:
Allows multi-line text input:
<label for="message">Your Message:</label>
<textarea id="message" name="message" rows="4" cols="50"></textarea>
5. Hidden Inputs:
Store data that isn’t visible to users:
<input type="hidden" name="user_id" value="12345">
Form Methods: GET vs POST
GET Method:
- Appends form data to the URL.
- Best for retrieving data or performing searches.
- Example:
<form action="search.php" method="get">
<input type="text" name="query" placeholder="Search...">
<input type="submit" value="Search">
</form>
POST Method:
- Sends data in the request body.
- Best for sensitive or large amounts of data.
- Example:
<form action="submit.php" method="post">
<input type="text" name="name" placeholder="Enter your name">
<input type="submit" value="Submit">
</form>
Validating Forms
Validation ensures that users provide accurate and complete data before submission. HTML5 provides built-in validation features:
Required Fields:
<input type="text" name="username" required>
Pattern Matching:
Use regular expressions for specific input patterns:
<input type="text" name="phone" pattern="[0-9]{10}" placeholder="10-digit phone number">
Email Validation:
<input type="email" name="email" required>
Number Validation:
<input type="number" name="age" min="18" max="99">
Enhancing Forms with CSS and JavaScript
Styling Forms with CSS:
You can use CSS to make forms visually appealing:
<style>
form {
max-width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
}
input, select, textarea {
width: 100%;
margin-bottom: 15px;
padding: 10px;
border: 1px solid #ccc;
border-radius: 3px;
}
</style>
Adding Interactivity with JavaScript:
JavaScript can validate form inputs dynamically:
<script>
document.querySelector("form").addEventListener("submit", function(event) {
const email = document.querySelector("#email").value;
if (!email.includes("@")) {
alert("Please enter a valid email address.");
event.preventDefault();
}
});
</script>
Best Practices for HTML Forms
- Use Labels for Accessibility: Labels improve usability, especially for screen readers.
- Group Related Inputs: Use
<fieldset>
and<legend>
to group related fields:<fieldset> <legend>Personal Information</legend> <label for="name">Name:</label> <input type="text" id="name" name="name"> </fieldset>
- Validate Both Client-Side and Server-Side: Client-side validation improves user experience, but always validate on the server for security.
- Keep Forms Simple: Avoid overwhelming users with too many fields.
Conclusion
HTML forms are a powerful tool for collecting and submitting user data. By understanding their structure and features, you can create functional and user-friendly forms for any website or application. As you build forms, remember to prioritize accessibility, validation, and user experience to ensure your forms are effective and reliable.